前回はSeekBarを表示する方法をまとめました。
今回は表示したSeekBarのデザインを変更してみましょう。
SeekBarの背景色を設定する
まずは背景の色を変更してみましょう
<SeekBar
android:id="@+id/seekBar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="#DDDDDD" <- SeekBarの背景色を変更する
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintBottom_toBottomOf="parent" />
// SeekBarのインスタンスを取得
SeekBar seekBar = findViewById(R.id.seekBar);
// SeekBarの背景色を変更する
seekBar.setBackgroundColor(Color.parseColor("#DDDDDD"));
// SeekBarのインスタンスを取得
val seekBar = findViewById<SeekBar>(R.id.seekBar)
// SeekBarの背景色を変更する
seekBar.setBackgroundColor(Color.parseColor("#DDDDDD"))
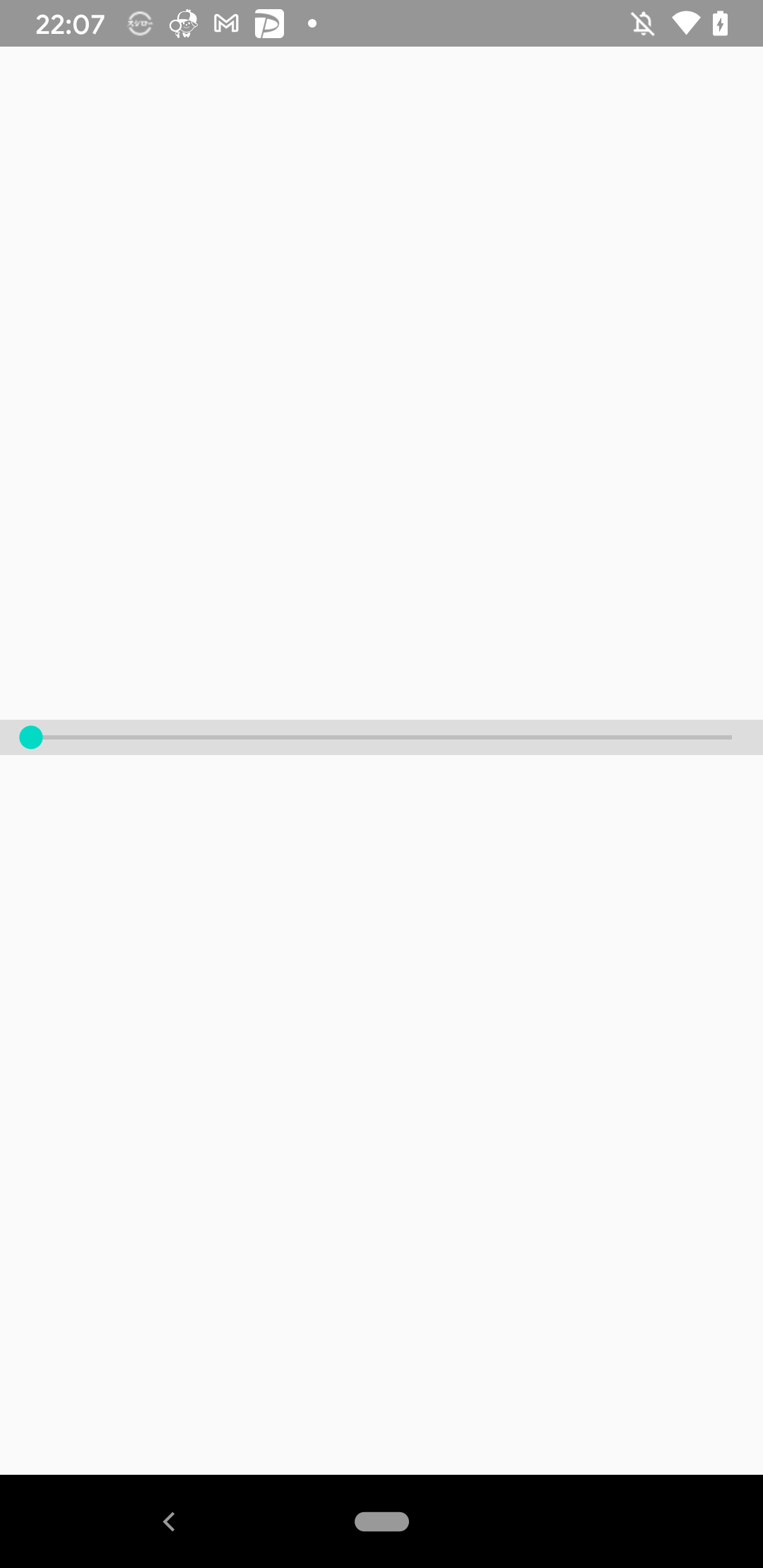
android:backgroundの説明
説明 | 例 | |
android:background | 指定したカラーコードに応じた色が背景色に設定される | #FF00000, #DDDDDD |
setBackgroundColor()の説明
引数型 | 例 | |
第一引数 | Int | 0xAARRGGBBの形式で定義して値に応じた色が背景色に設定される |
SeekBarのつまみの色を変更する
続いてはつまみの色を変更しましょう
デフォルトではres/colors.xmlに記載しているcolorAccentの色が反映されます。
<SeekBar
android:id="@+id/seekBar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:thumbTint="#FF0000" <- SeekBarのつまみの色を変更する
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintBottom_toBottomOf="parent" />
// SeekBarのインスタンスを取得
SeekBar seekBar = findViewById(R.id.seekBar);
// SeekBarのつまみの色を変更する
seekBar.getThumb().setTintList(ColorStateList.valueOf(Color.parseColor("#FF0000")));
// SeekBarのインスタンスを取得
val seekBar = findViewById<SeekBar>(R.id.seekBar)
// SeekBarのつまみの色を変更する
seekBar.thumb.setTintList(ColorStateList.valueOf(Color.parseColor("#FF0000")))}
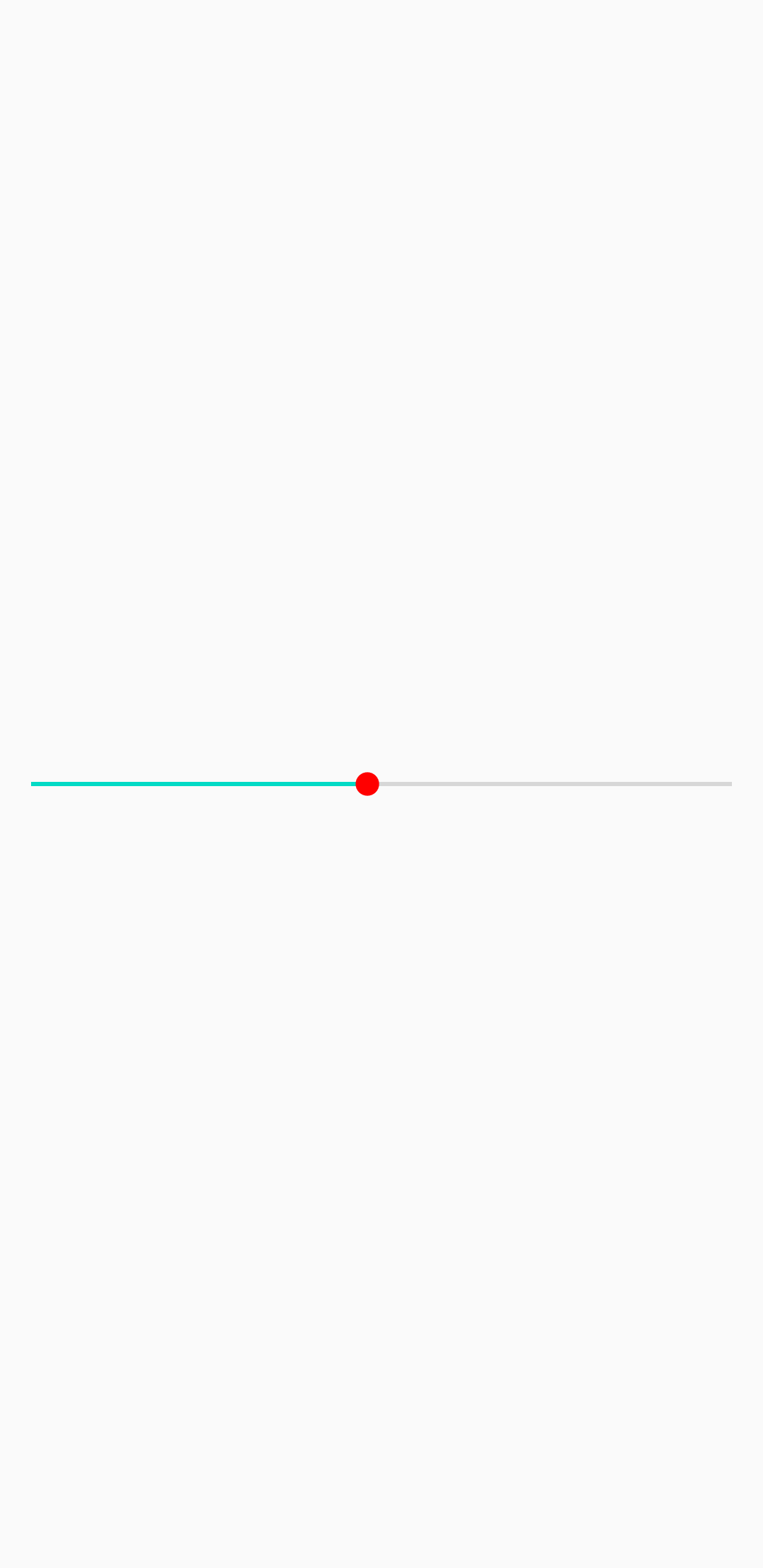
android:thumbTintの説明
説明 | 例 | |
android:thumbTint | 設定したカラーコードがつまみの色に反映される | #FF0000, #DDFF0000 |
getThumb()の説明
戻り値 | 説明 |
Drawable | つまみの描画に使用しているDrawableが返却される |
Drawable = 描画用のリソース
setTintList()の説明
引数型 | 説明 | |
第一引数 | ColorStateList | 描画に使用するColorStateListを設定する |
ColorStateList = 状態によったカラーを定義する
選択中や非活性時の色を設定できます。
SeekBarのバーの色を変更する
ではバーの色を変更しましょう
<SeekBar
android:id="@+id/seekBar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:progressBackgroundTint="#FF0000" <- SeekBarのバーの色を変更する
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintBottom_toBottomOf="parent" />
// SeekBarのインスタンスを取得
SeekBar seekBar = findViewById(R.id.seekBar);
// SeekBarのバーの色を変更する
seekBar.setProgressBackgroundTintList(ColorStateList.valueOf(Color.parseColor("#FF0000")));
// SeekBarのインスタンスを取得
val seekBar = findViewById<SeekBar>(R.id.seekBar)
// SeekBarのバーの色を変更する
seekBar.progressBackgroundTintList = ColorStateList.valueOf(Color.parseColor("#FF0000"))
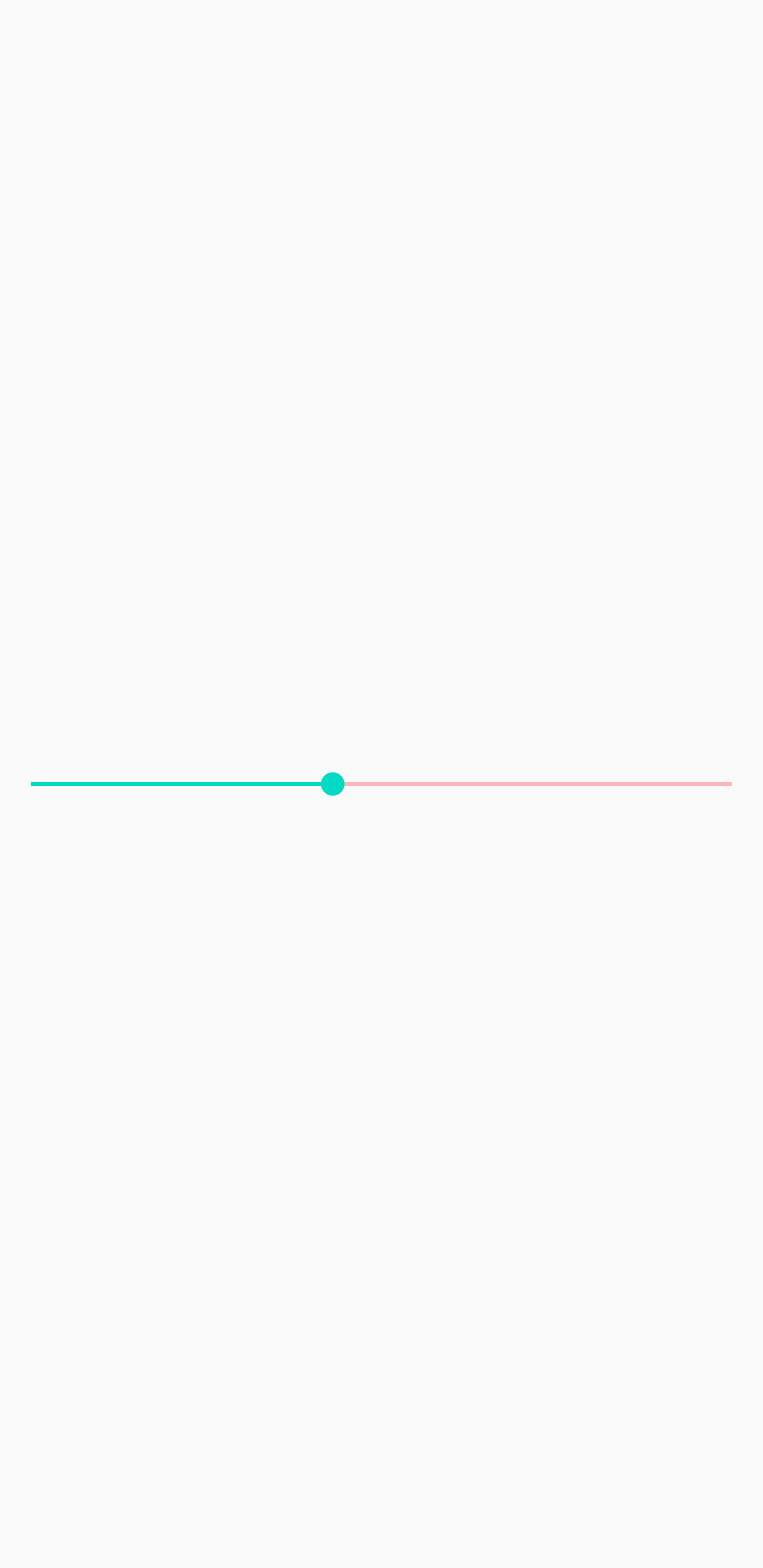
android:progressBackgroundTintの説明
説明 | 例 | |
android:thumbTint | 設定したカラーコードがバーの色に反映される | #FF0000, #DDFF0000 |
setProgressBackgroundTintListの説明
引数型 | 説明 | |
第一引数 | ColorStateList | バーの描画に使用するColorStateListを設定する |
SeekBarの進捗バーの色を変更する
次は進捗バーの色を変更しましょう
デフォルトの色はつまみ同様res/colors.xmlに記載しているcolorAccentです。
<SeekBar
android:id="@+id/seekBar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:progressTint="#FF0000" <- SeekBarの進捗バーの色を変更する
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintBottom_toBottomOf="parent" />
// SeekBarのインスタンスを取得
SeekBar seekBar = findViewById(R.id.seekBar);
// SeekBarの進捗バーの色を変更する
seekBar.setProgressTintList(ColorStateList.valueOf(Color.parseColor("#FF0000")));
// SeekBarのインスタンスを取得
val seekBar = findViewById<SeekBar>(R.id.seekBar)
// SeekBarの進捗バーの色を変更する
seekBar.progressTintList = ColorStateList.valueOf(Color.parseColor("#FF0000"))
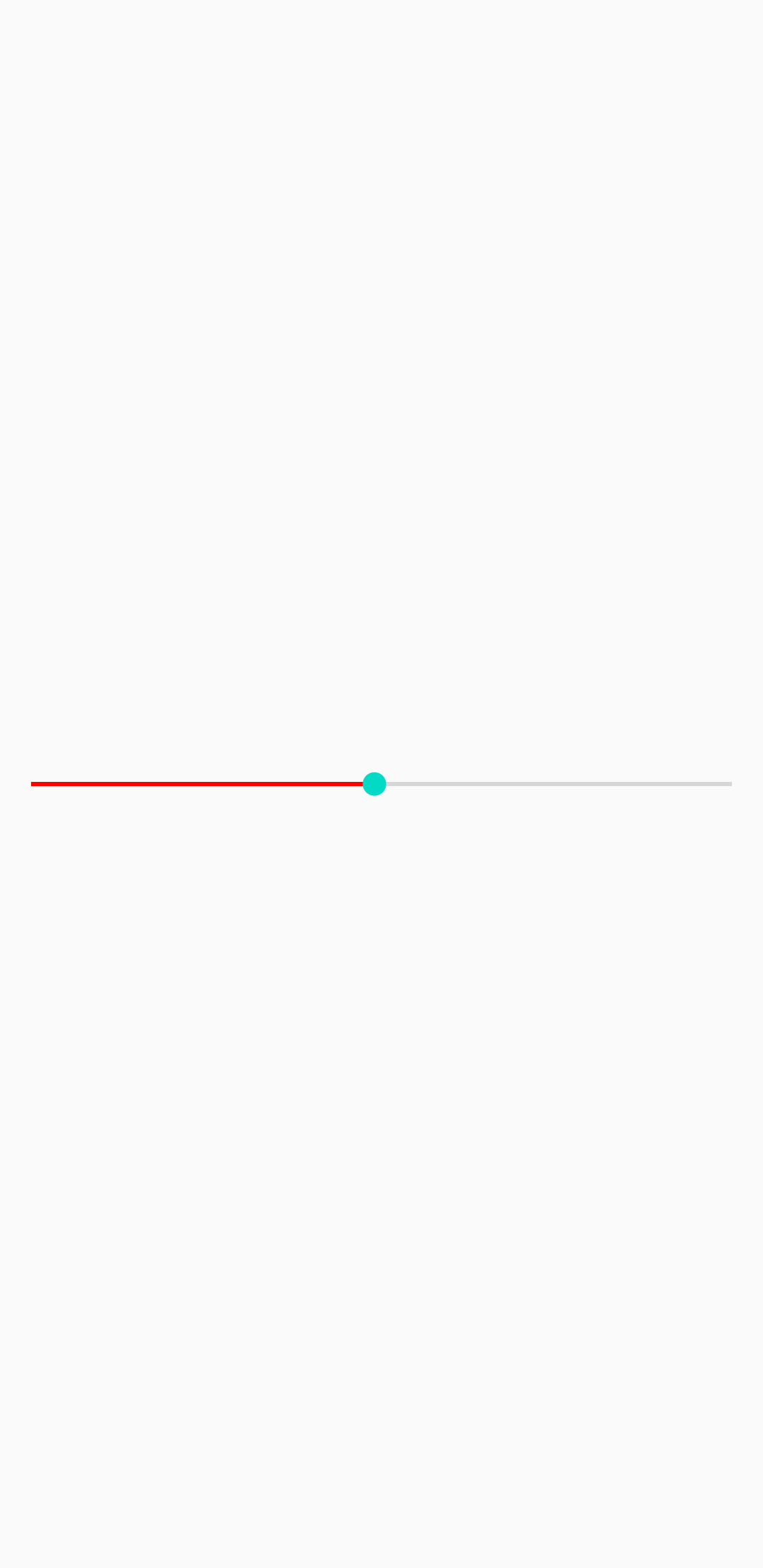
android:progressTintの説明
説明 | 例 | |
android:progressTint | 設定したカラーコードが進捗バーの色に反映される | #FF0000, #DDFF0000 |
setProgressTintList()の説明
引数型 | 説明 | |
第一引数 | ColorStateList | 進捗バーの描画に使用するColorStateListを設定する |
SeekBarのセカンダリプログレスバーの色を変更する
最後にセカンダリプログレスバーの色を変更しましょう
セカンダリプログレスバーといえばYoutubeで言うところの動画読み込み済みを示すあのバーですね!
<SeekBar
android:id="@+id/seekBar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:secondaryProgressTint="#FF0000" <- SeekBarのセカンダリプログレスバーの色を変更する
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintBottom_toBottomOf="parent" />
// SeekBarのインスタンスを取得
SeekBar seekBar = findViewById(R.id.seekBar);
// SeekBarのセカンダリプログレスバーの色を変更する
seekBar.setSecondaryProgressTintList(ColorStateList.valueOf(Color.parseColor("#FF0000")));
// SeekBarのインスタンスを取得
val seekBar = findViewById<SeekBar>(R.id.seekBar)
// SeekBarのセカンダリプログレスバーの色を変更する
seekBar.secondaryProgressTintList = ColorStateList.valueOf(Color.parseColor("#FF0000"))
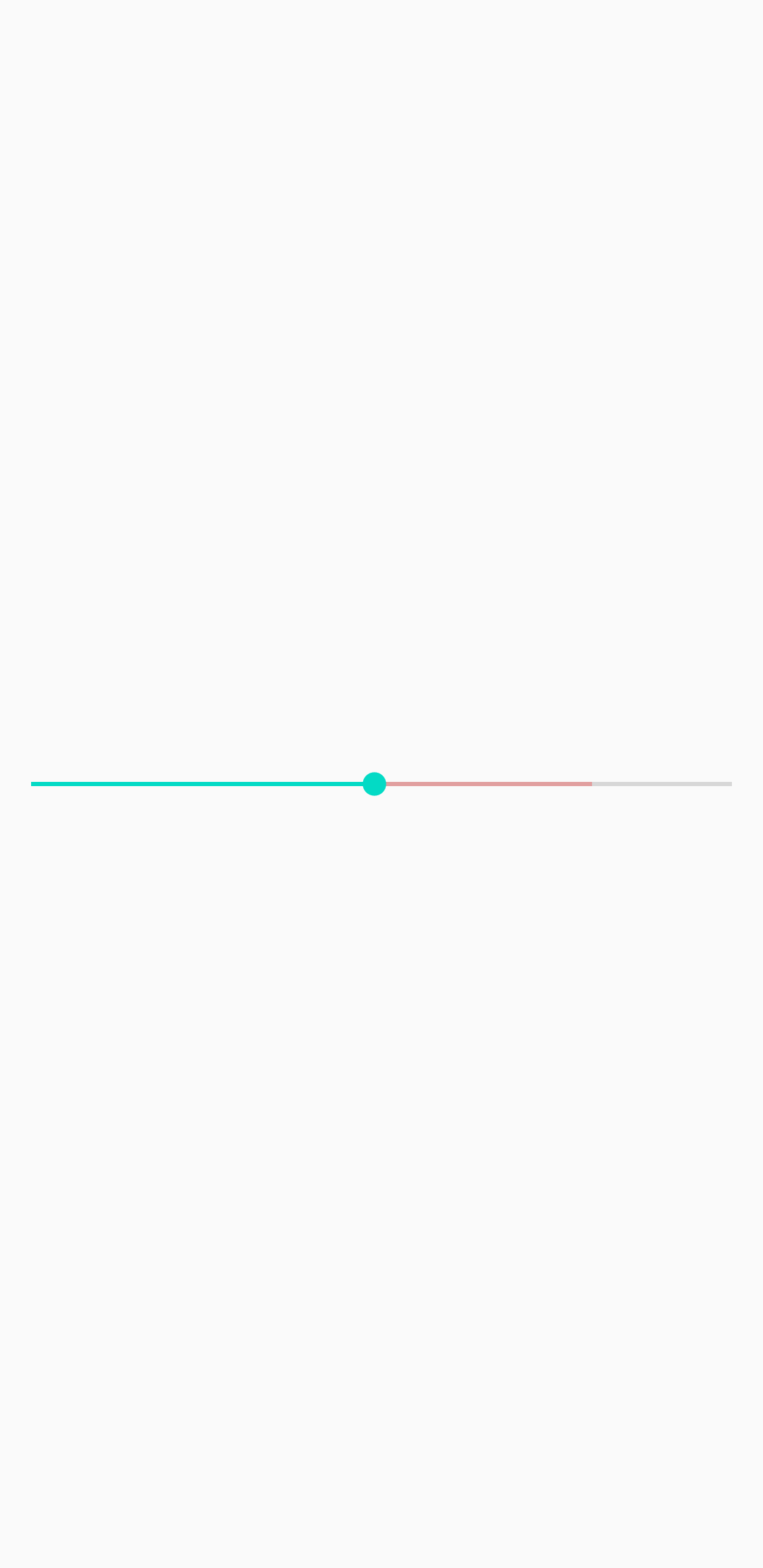
android:secondaryProgressTintの説明
説明 | 例 | |
android:secondaryProgressTint | 設定したカラーコードがセカンダリプログレスバーの色に反映される | #FF0000, #DDFF0000 |
setSecondaryProgressTintList()の説明
引数型 | 説明 | |
第一引数 | ColorStateList | セカンダリプログレスバーの描画に使用するColorStateListを設定する |
最後に
いかがでしたでしょうか
今回まとめた事を同時に使用する事で
オリジナルのSeekBarになるのではないでしょうか
コメント